STF多设备管理平台
https://github.com/openstf
部署
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| $IP #拉取openstf镜像 docker pull openstf/stf:latest #拉取adb镜像 docker pull sorccu/adb:latest #拉取ambassador镜像 docker pull openstf/ambassador:latest #拉取rethinkdb数据库镜像
docker pull rethinkdb:latest #拉取nginx镜像 docker pull nginx:latest #启动数据库 docker run -d --name rethinkdb -v ~/docker/STF/rethinkdb:/data --net host rethinkdb rethinkdb --bind all --cache-size 8192 --http-port 8090 #启动adb service docker run -d --name adbd --privileged -v /dev/bus/usb:/dev/bus/usb/ --net host sorccu/adb:latest
#启动stf服务 docker run -d --name stf --net host openstf/stf stf local --public-ip $IP
#nodaemon模式启动远端机器adb server并对外暴露5037端口 adb nodaemon server -a -P 5037
#通过配置 adb host 的方式连上远端机器的设备 stf provider --name centos7 --min-port 7400 --max-port 7700 --connect-sub tcp://127.0.0.1:7114 --connect-push tcp://127.0.0.1:7116 --group-timeout 900 --public-ip 192.168.13.128 --storage-url http://localhost:7100/ --adb-host 192.168.13.1 --adb-port 5037 --vnc-initial-size 600x800 --mute-master never --allow-remote #如要连装stf服务的机器的设备,不需要增加--adb-host stf provider --name centos --min-port 7400 --max-port 7700 --connect-sub tcp://127.0.0.1:7114 --connect-push tcp://127.0.0.1:7116 --group-timeout 20000 --public-ip 192.168.13.128 --storage-url http://localhost:7100/ --vnc-initial-size 600x800 --allow-remote
|
stf服务搭建脚本
https://github.com/guobq/mysource/blob/master/dockerbuildSTF.sh
操作可参考:
https://testerhome.com/topics/7966?locale=en
https://blog.csdn.net/FloatDreamed/article/details/103809814?utm_medium=distribute.pc_relevant_t0.none-task-blog-BlogCommendFromMachineLearnPai2-1.channel_param&depth_1-utm_source=distribute.pc_relevant_t0.none-task-blog-BlogCommendFromMachineLearnPai2-1.channel_param
非交互式调用
https://github.com/openstf/stf/blob/master/doc/API.md
1 2 3 4 5 6 7 8 9
| curl -H "Authorization: Bearer YOUR-TOKEN-HERE" https://stf.example.org/api/v1/user # 获得设备列表: curl -H "Authorization: Bearer $key" http://localhost:7100/api/v1/devices | jq .devices[].serial # 申请设备 curl -X POST --header "Content-Type: application/json" --data '{"serial":"$device"}' -H "Authorization: Bearer $key" http://127.0.0.1:7100/api/v1/user/devices # 远程调试 curl -X POST -H "Authorization: Bearer $key" http://127.0.0.1:7100/api/v1/user/devices/$device/remoteConnect # 释放设备 curl -X DELETE -H "Authorization: Bearer $key" http://127.0.0.1:7100/api/v1/user/devices/$device
|
adb 架构
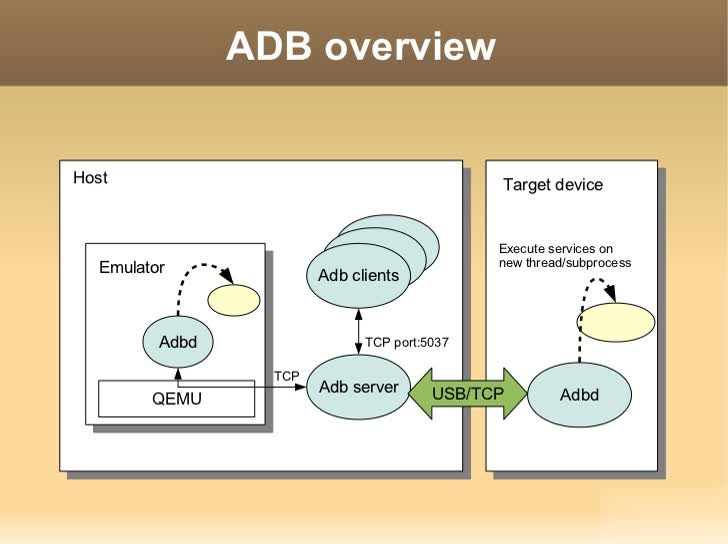
shell实现方式(依赖 jq)
jq:https://stedolan.github.io/jq/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74
| #!/usr/bin/env bash
set -euxo pipefail STF_URL=http://192.168.13.128:7100/ STF_TOKEN=
if [ "$DEVICE_SERIAL" == "" ]; then echo "Please specify device serial using ENV['DEVICE_SERIAL']" exit 1 fi
if [ "$STF_URL" == "" ]; then echo "Please specify stf url using ENV['STF_URL']" exit 1 fi
if [ "$STF_TOKEN" == "" ]; then echo "Please specify stf token using using ENV['STF_TOKEN']" exit 1 fi
function add_device { response=$(curl -X POST -H "Content-Type: application/json" \ -H "Authorization: Bearer $STF_TOKEN" \ --data "{\"serial\": \"$DEVICE_SERIAL\"}" $STF_URL/api/v1/user/devices)
success=$(echo "$response" | jq .success | tr -d '"') description=$(echo "$response" | jq .description | tr -d '"')
if [ "$success" != "true" ]; then echo "Failed because $description" exit 1 fi
echo "Device $DEVICE_SERIAL added successfully" }
function remote_connect { response=$(curl -X POST \ -H "Authorization: Bearer $STF_TOKEN" \ $STF_URL/api/v1/user/devices/$DEVICE_SERIAL/remoteConnect)
success=$(echo "$response" | jq .success | tr -d '"') description=$(echo "$response" | jq .description | tr -d '"')
if [ "$success" != "true" ]; then echo "Failed because $description" exit 1 fi remote_connect_url=$(echo "$response" | jq .remoteConnectUrl | tr -d '"')
adb connect $remote_connect_url
echo "Device $DEVICE_SERIAL remote connected successfully" }
function remove_device { response=$(curl -X DELETE \ -H "Authorization: Bearer $STF_TOKEN" \ $STF_URL/api/v1/user/devices/$DEVICE_SERIAL)
success=$(echo "$response" | jq .success | tr -d '"') description=$(echo "$response" | jq .description | tr -d '"')
if [ "$success" != "true" ]; then echo "Failed because $description" exit 1 fi
echo "Device $DEVICE_SERIAL removed successfully" }
|
jenkins集成业务逻辑
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| # 获取设备uuid,厂家,os版本,屏幕尺寸 adb devices | grep device | grep -v List |awk '{print $1}' | while read line;do brand=$(adb -s $line shell getprop ro.product.manufacturer);osversion=$(adb -s $line shell getprop ro.build.version.release);size=$(adb -s $line shell wm size);echo $line $brand $osversion $size;done # jenkins执行远程shell echo brand=$brand os=$os size=$size grep -1 "$brand.*$os." / tmp/devices device=$(grep -i "$brand.*$os.*" /tmp/devices| awk '{print $1}') adb -s $device shell wm size # emulator-5554 Google 10 Physical size: 1440x2560 DEVICE_SERIAL=$device # 上面shell实现方式,可以以sh脚本方式调用:.stf.sh # 业务逻辑 add_device remote_connect sleep 2 adb_devices # 执行脚本 echo run monkey or appcrawler adb -s $remote_connect_url shell monkey --throttle -p com.android.calculator2 200 remove_device adb devices adb disconnect
|